Design Pattern
Mediator/Middleware Pattern
The mediator pattern makes it possible for components to interact with each other through a central point: the mediator. Instead of directly talking to each other, the mediator receives the requests, and sends them forward! In JavaScript, the mediator is often nothing more than an object literal or a function.
You can compare this pattern to the relationship between an air traffic controller and a pilot. Instead of having the pilots talk to each other directly, which would probably end up being quite chaotic, the pilots talk the air traffic controller. The air traffic controller makes sure that all planes receive the information they need in order to fly safely, without hitting the other airplanes.
Although we’re hopefully not controlling airplanes in JavaScript, we often have to deal with multidirectional data between objects. The communication between the components can get rather confusing if there is a large number of components.
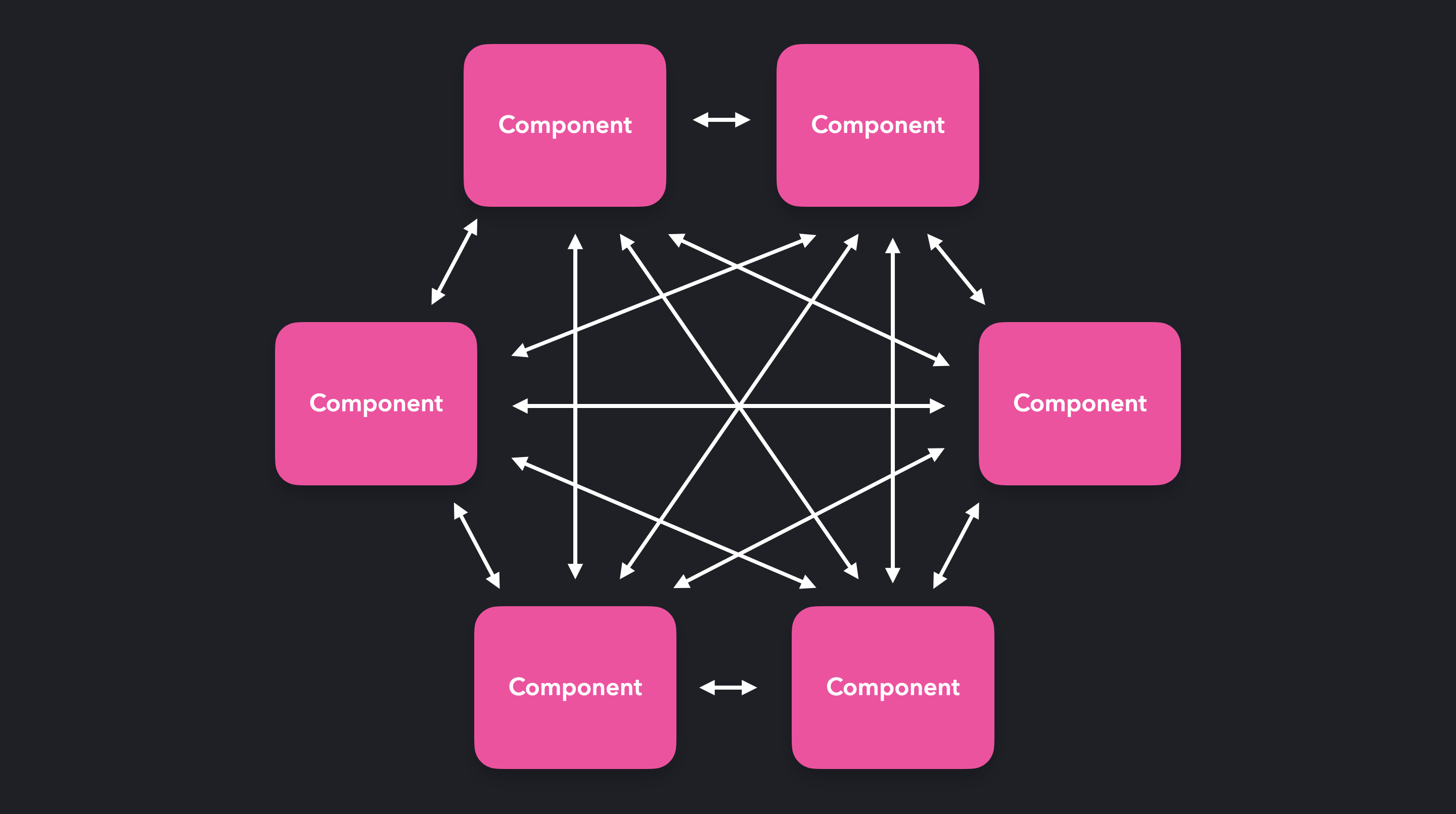
Instead of letting every objects talk directly to the other objects, resulting in a many-to-many relationship, the object’s requests get handled by the mediator. The mediator processes this request, and sends it forward to where it needs to be.
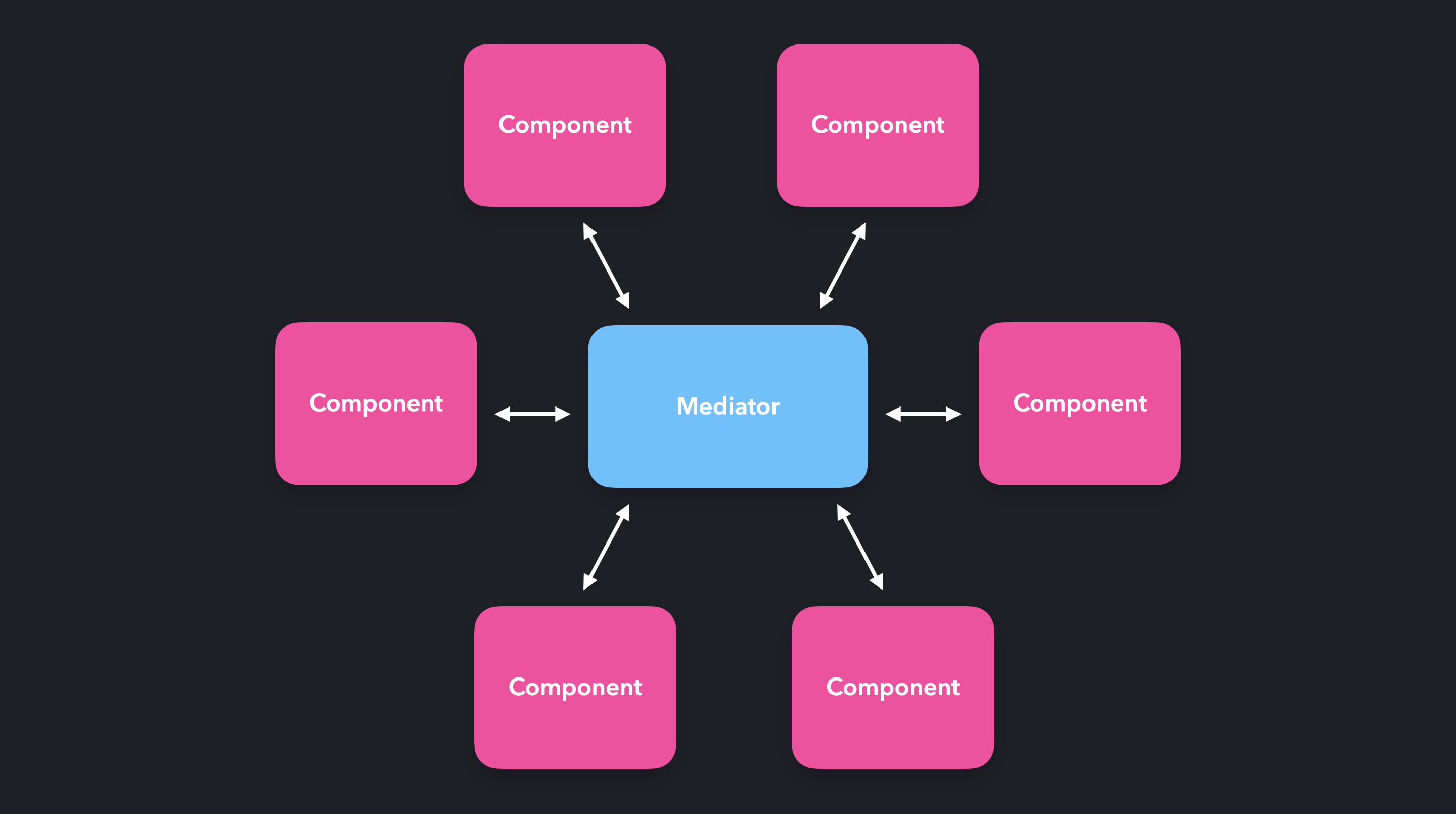
A good use case for the mediator pattern is a chatroom! The users within the chatroom won’t talk to each other directly. Instead, the chatroom serves as the mediator between the users.
class ChatRoom {
logMessage(user, message) {
const time = new Date();
const sender = user.getName();
console.log(`${time} [${sender}]: ${message}`);
}
}
class User {
constructor(name, chatroom) {
this.name = name;
this.chatroom = chatroom;
}
getName() {
return this.name;
}
send(message) {
this.chatroom.logMessage(this, message);
}
}
We can create new users that are connected to the chat room. Each user instance has a send
method which we can use in order to send messages.
1class ChatRoom {2 logMessage(user, message) {3 const sender = user.getName();4 console.log(`${new Date().toLocaleString()} [${sender}]: ${message}`);5 }6}78class User {9 constructor(name, chatroom) {10 this.name = name;11 this.chatroom = chatroom;12 }1314 getName() {15 return this.name;16 }1718 send(message) {19 this.chatroom.logMessage(this, message);20 }21}2223const chatroom = new ChatRoom();2425const user1 = new User("John Doe", chatroom);26const user2 = new User("Jane Doe", chatroom);2728user1.send("Hi there!");29user2.send("Hey!");
Case Study
Express.js is a popular web application server framework. We can add callbacks to certain routes that the user can access.
Say we want add a header to the request if the user hits the root '/'
. We can add this header in a middleware callback.
const app = require("express")();
app.use("/", (req, res, next) => {
req.headers["test-header"] = 1234;
next();
});
The next
method calls the next callback in the request-response cycle. We’d effectively be creating a chain of middleware functions that sit between the request and the response, or vice versa.
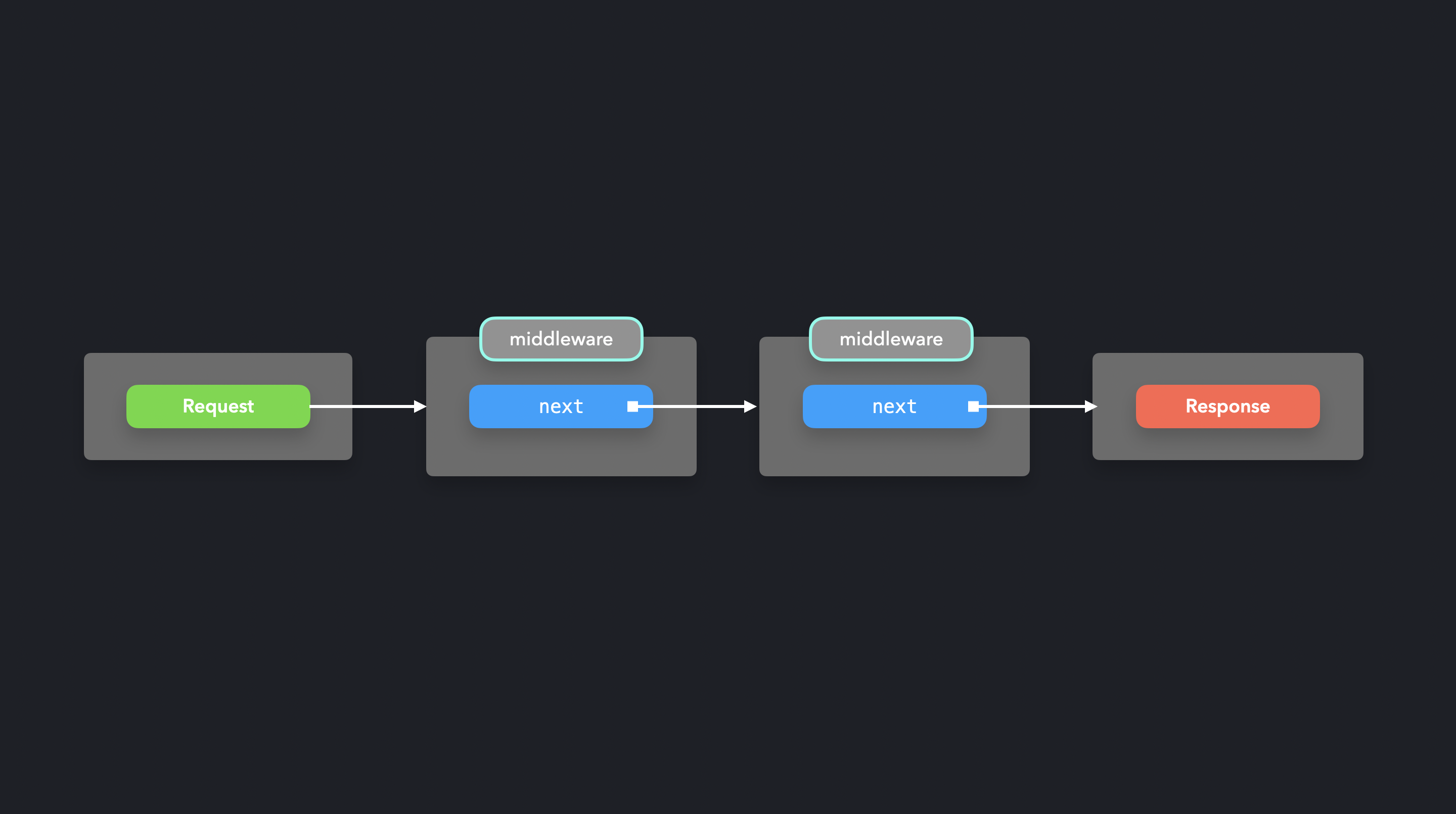
Let’s add another middleware function that checks whether the test-header
was added correctly. The change added by the previous middleware function will be visible throughout the chain.
const app = require("express")();
app.use(
"/",
(req, res, next) => {
req.headers["test-header"] = 1234;
next();
},
(req, res, next) => {
console.log(`Request has test header: ${!!req.headers["test-header"]}`);
next();
}
);
Perfect! We can track and modify the request object all the way to the response through one or multiple middleware functions.
1const app = require("express")();2 const html = require("./data");34 app.use(5 "/",6 (req, res, next) => {7 req.headers["test-header"] = 1234;8 next();9 },10 (req, res, next) => {11 console.log(`Request has test header: ${!!req.headers["test-header"]}`);12 next();13 }14 );1516 app.get("/", (req, res) => {17 res.set("Content-Type", "text/html");18 res.send(Buffer.from(html));19 });2021 app.listen(8080, function() {22 console.log("Server is running on 8080");23 });
Every time the user hits a root endpoint '/'
, the two middleware callbacks will be invoked.
The middleware pattern makes it easy for us to simplify many-to-many relationships between objects, by letting all communication flow through one central point.